Loops in Python
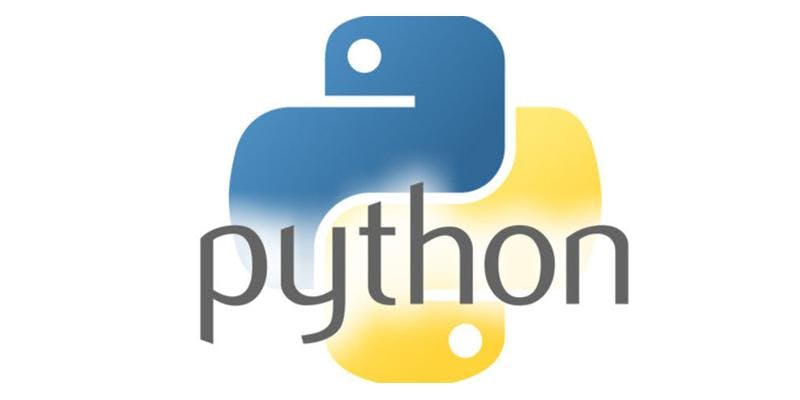
Loops are important in Python or in any other programming language as they help you to execute a block of code repeatedly. You will often come face to face with situations where you would need to use a piece of code over and over but you don't want to write the same line of code multiple times.
In general, statements are executed sequentially: The first statement in a function is executed first, followed by the second, and so on. There may be a situation when you need to execute a block of code several number of times. Loops are important in Python or in any other programming language because they help you to execute a block of code repeatedly. You will be faced with situations where you will need to use a piece of code over and over but you don't want to write the same line of code multiple times. In Python, there are two types of loops, for and while.
For Loop
The for loop that is used to iterate over elements of a sequence, it is often used when you have a piece of code which you want to repeat "n" number of time. For loops can iterate over a sequence of numbers using the range and xrange functions. The difference between range and xrange is that the range function returns a new list with numbers of that specified range, whereas xrange returns an iterator, which is more efficient. The range function is zero based. If you want to write code that will run on both Python 2 and Python 3, use range() as the xrange funtion is deprecated in Python 3.
for x in range(5): print(x)
Prints out the numbers 0,1,2,3,4
for x in range(3, 6): print(x)
Prints out 3,4,5
While Loop
A while loop executes some of code over and over again while a given condition still holds true. While loops need three components that consists of the while keyword, a condition that evaluates to True or False and a block of code that we want to execute repeatedly. Unlike a for loop, where execution of the code block will stop after a set number of iterations( or earlier if a break statement is used ), a while loop may be infinite.
count = 1 while count < 5: print(count) count += 1
Will print out 1, 2, 3 , 4.
control = 0 count = 1 while control < 5: print(count) count += 1
Will print out 1, 2, 3 , 4 . . . and never exit the loop.
Control Statements
Loop control statements change execution from its normal sequence. When execution leaves a scope, all automatic objects that were created in that scope are destroyed. The break statement terminates the loop statement and transfers execution to the statement immediately following the loop. The continue statement causes the loop to skip the remainder of its body and immediately retest its condition prior to reiterating. The pass statement is used when a statement is required syntactically but you do not want any command or code to execute.
count = 1 while True: print(count) count += 1 if count >= 5: break
Prints out 1, 2, 3, 4.
for x in range(10): if x % 2 == 0: continue print(x)
Prints out only odd numbers, 1, 3, 5, 7, 9.
Unlike languages like C,C++, we can use else for loops. When the loop condition of a for or while statement fails then code part in else is executed. If break statement is executed inside for loop then the else part is skipped. The else part is executed even if there is a continue statement.