Variable scope in Java
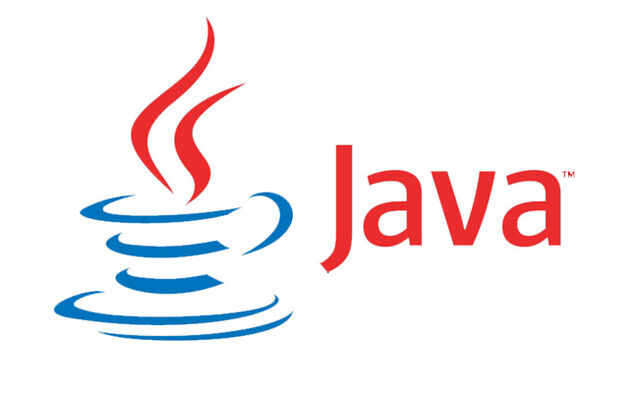
Knowing when you can use a variable gets confusing if you don't understand variable scope. The scope of a variable is the part of the program where the variable is accessible. Java programs are organized in the form of classes. Every class is part of some package. As a general rule, variables that are defined within a block are not accessible outside that block. The lifetime of a variable refers to how long the variable exists before it is destroyed. Destroying variables refers to deallocating the memory that was allotted to the variables when declaring it. Many other computer languages defines two general categories of scopes: global and local. However, these traditional scopes do not fit well with Java's strict, object-oriented model. While it is possible to mimic a global scope, it is the exception, not the rule.
Scoping rules
As a rule, variables declared inside a scope are not visible or accessible to the code that is defined outside the scope. When we declare a variable within a scope, we are localizing that variable and protecting it from an unauthorized access and modification. The scope rules provide the foundation for encapsulation. Scopes can be nested. For example, each time we create a block of code, we are creating a new, nested scope. When this occurs, the outer scope encloses the inner scope. What this means is that the objects declared in the outer scope will be visible to code within the inner scope but the reverse is not true. Objects declared within the inner scope will not be visible outside it.
1 public class ScopeExample 2 { 3 public static void main( String args[] ) 4 { 5 int x; 6 7 x = 10; 8 if(x == 10) 9 { 10 int y = 20; 11 12 System.out.println("x : " + x + " y : " + y); 13 x = y * 2; 14 } 15 16 System.out.println("x is " +x); 17 // System.out.println("y = " + y ) ; 18 } 19 }
The variable x can be seen in the if statement but the variable y can't be seen in the main scope.
Class scope
In Java, there are some variables that we want to be able to access from anywhere within a Java class. The scope of these variables will need to be at the class level, and there is only one way to create variables at that level. These variables must be declared inside class and outside any function. They can be directly accessed anywhere in class. Member variables can be accessed outside a class using access identifiers. The access identifier does not affect the variable's scope within the class. Below are some access identifiers and access rules:
Modifier Package Subclass Everywhere
public yes yes yes protected yes yes no no modifier yes no no private no no no
Method scope
Variables declared inside a method have method level scope and can't be accessed outside the method. When the method ends, the variable reference goes away and there is no way to access that variable any longer. Method level scope variables can be declared in the parameter list of the method declaration. We can use the same variable name as a class scoped variable but we must use the this. prefix to distinguish it from the class variable.
1 public class Test 2 { 3 private int y; 4 5 void method1() 6 { 7 int x; 8 } 9 10 void sety( int y ) 11 { 12 this.y = 100 ; 13 } 14 }
In line 7, we declare a variable x that is only available inside method1. In line 10, we declare a variable y that is only accessible in sety method and we use the this. to distinguish the method variable y from the class variable y.
Block scope
A variable declared inside pair of curly brackets in a method has scope within the brackets only. Once we exit the loop, the variable is no longer accessible. This includes any variables created in the loop signature. In Java, a variable declared in the block scope can't have the same name as a method scoped variable and will give an error unlike C++ where the compiler tracks the scope.
Block scoped variables x and y
class Test { public static void main(String args[]) { { int y = 10; System.out.println(y); } for (int x = 0; x < 4; x++) { System.out.println(x); } } }
Method scoped variable x
class Test { public static void main(String args[]) { int x; for (x = 0; x < 4; x++) { System.out.println(x); } System.out.println(x); } }
Block scope variable name is the same as method variable name. Will produce error in Java but will run in C++
class Test { public static void main(String args[]) { int a = 5; for (int a = 0; a < 5; a++) { System.out.println(a); } } }